What is Popup in Angular?
In Angular, a “popup” is like a small window suddenly appearing on your web page.
Angular makes it easy to create popups through libraries that offer pre-designed components and tools.
With Angular, you can create various types of popups as follows.
Modal Dialogues: You can use Angular Material's ‘MatDialog’ module.
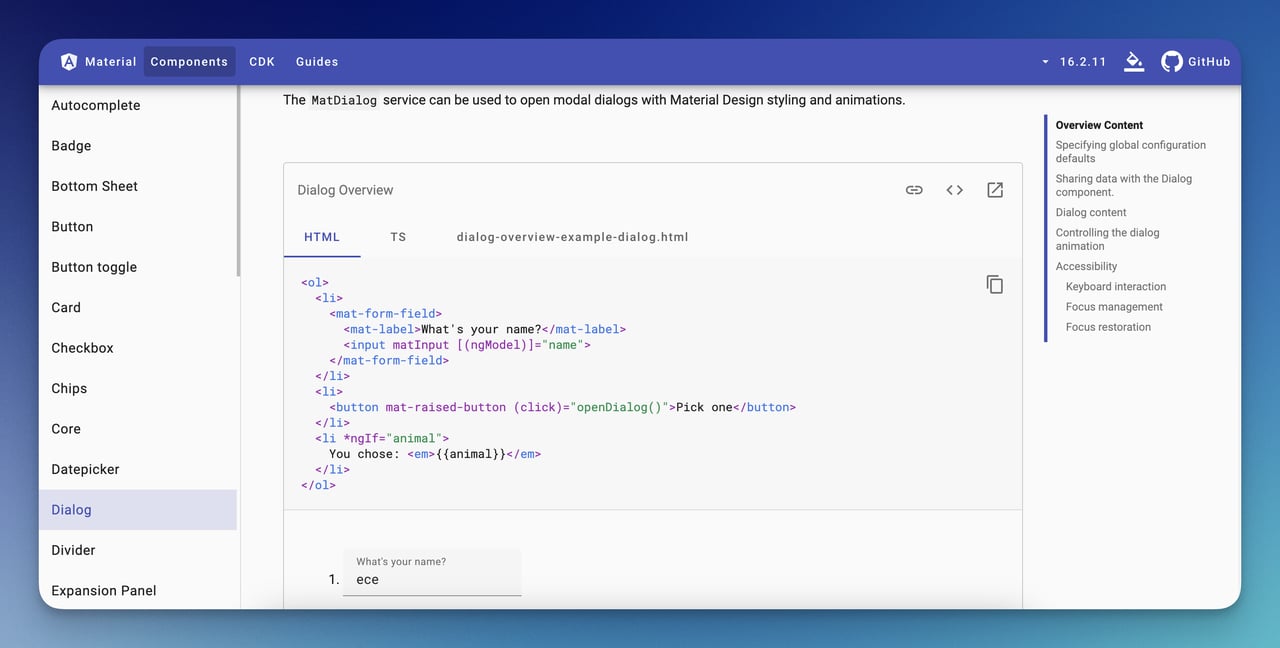
They are popups that overlay the main content of a page.
Toasts/Notifications: You can use Angular Material's ‘MatSnackBar’ component.
It is a type of popup that appears briefly to show messages or warnings and disappears after.
Popups/ToolTips: You can use Angular's ngIf, ngClass, and event bindings.
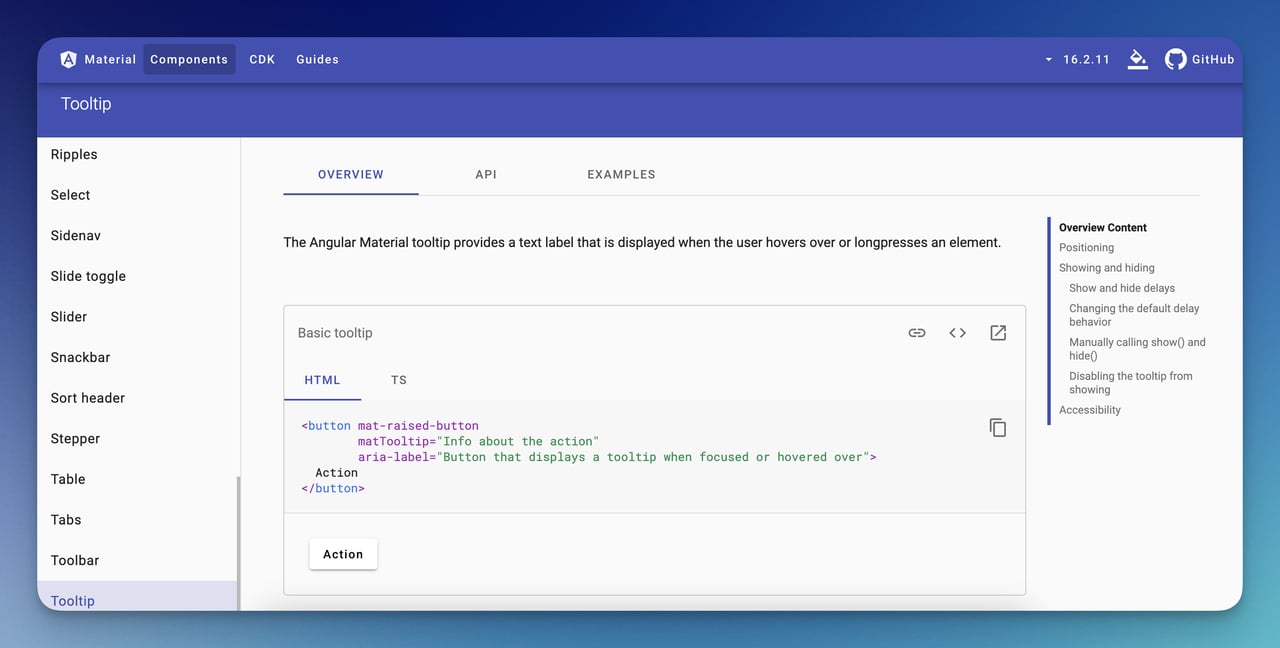
They are small pop-ups that provide additional information or context when the user hovers over a button or link.
Drop-down Menus: You can use Angular directives (e.g., ngIf, ngFor) and custom components.
Accordions: You can use Angular directives and custom components.
Side Panels: You can create them by defining a separate route in Angular's routing configuration.
4 Ways to Create Popup in Angular

Check out these step-by-step instructions on how to create popups using Angular Materials, Ng-bootstrap, PrimeNG, and Popupsmart:
Among these methods, you can choose the one that best suits your flexibility and customization needs:
1. Creating a Popup in Angular With Angular Materials
To create a popup in Angular using Angular Materials, you can use the MatDialog module.
Step 1: Install Angular Materials.
Open your terminal and run the following command:
Step 2: Create a popup component.
You can use the Angular CLI to generate it:
Step 3: Define the popup content.
Edit the ‘popup.component.html’ file to define the content of your popup:
Step 4: Create a service
Create a service to handle the opening and closing of the popup.
Step 5: Edit the ‘popup.service.ts’ file.
Step 6: Use the popup service in your component.
Import the PopupService and use it to open the popup when a button is clicked.
Step 7: Import the MatDialog Module.
Last Step: Run your popup.
2. Creating a Popup in Angular With Ng-bootstrap
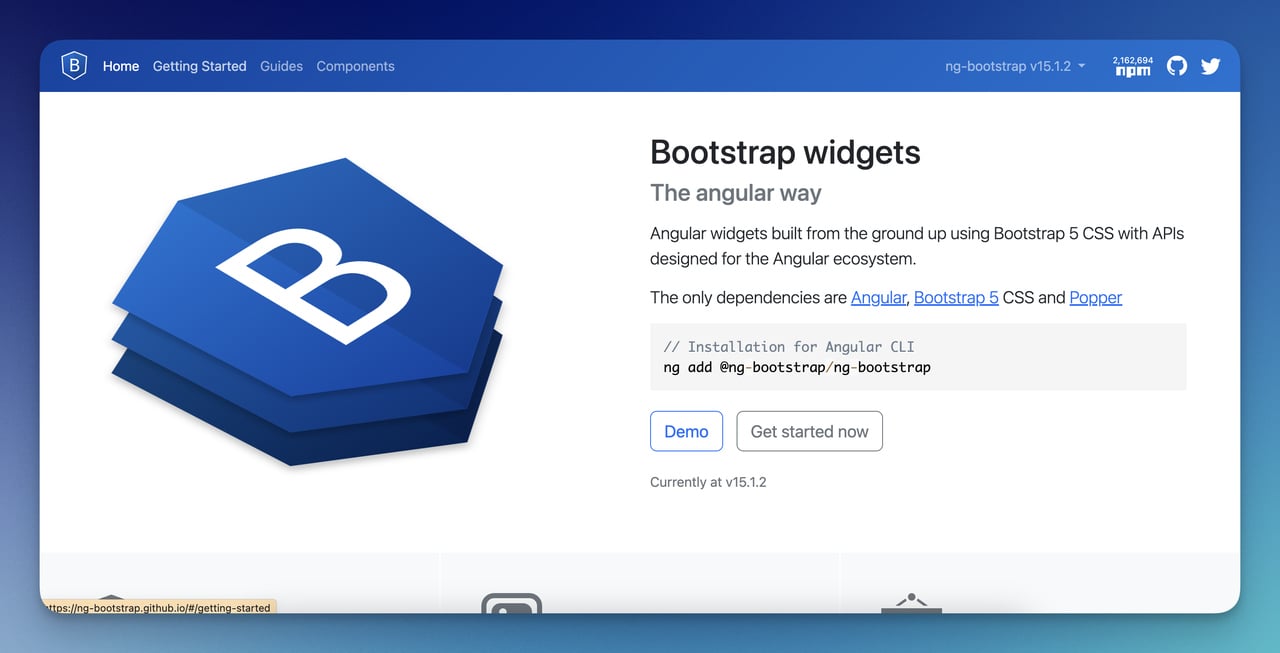
To create a popup in Angular using Ng-bootstrap, you can use the NgbModal module.
Step 1: Install Ng-bootstrap.
Step 2: Generate a component for your popup.
Step 3: Import NgbModal from Ng-bootstrap.
Step 4: Create a component for the popup.
Step 5: Open the popup component.
Step 6: Create a button to trigger the popup.
Step 7: Create the popup functionality.
Last Step: Run your popup.
3. Creating a Popup in Angular With PrimeNG
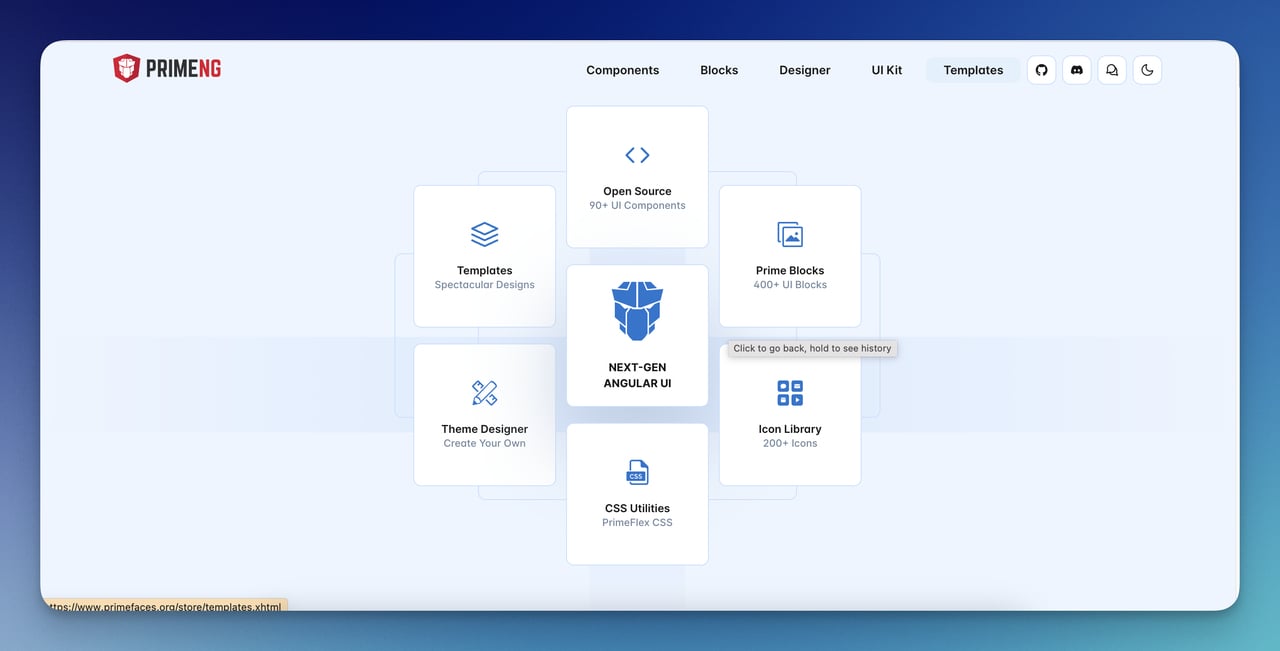
To create a popup in Angular with PrimeNG, you can use the p-dialog component.
Step 1: Install PrimeNG and its required dependencies.
Step 2: Import PrimeNG Styles.
Step 3: Import DialogModule.
Step 4: Create the popup component.
Import { Component } from '@angular/core';
Step 5: Use the dialog component.
Last Step: Run your popup.
Note: Creating popups in Angular with various UI libraries like Angular Materials, Ng-bootstrap, and PrimeNG involves nearly identical steps, with minor differences in library-specific language and configuration.
And here is the bonus step 🌟
4. Creating a Popup in Angular with Popupsmart
If you don't have advanced development skills or time to code for creating popups in Angular, it might be wise to use a no-code popup builder like Popupsmart.
After signing up for Popupsmart, you can choose the pop-up that suits your style from the playbook and then publish it on your Angular site. That's it!
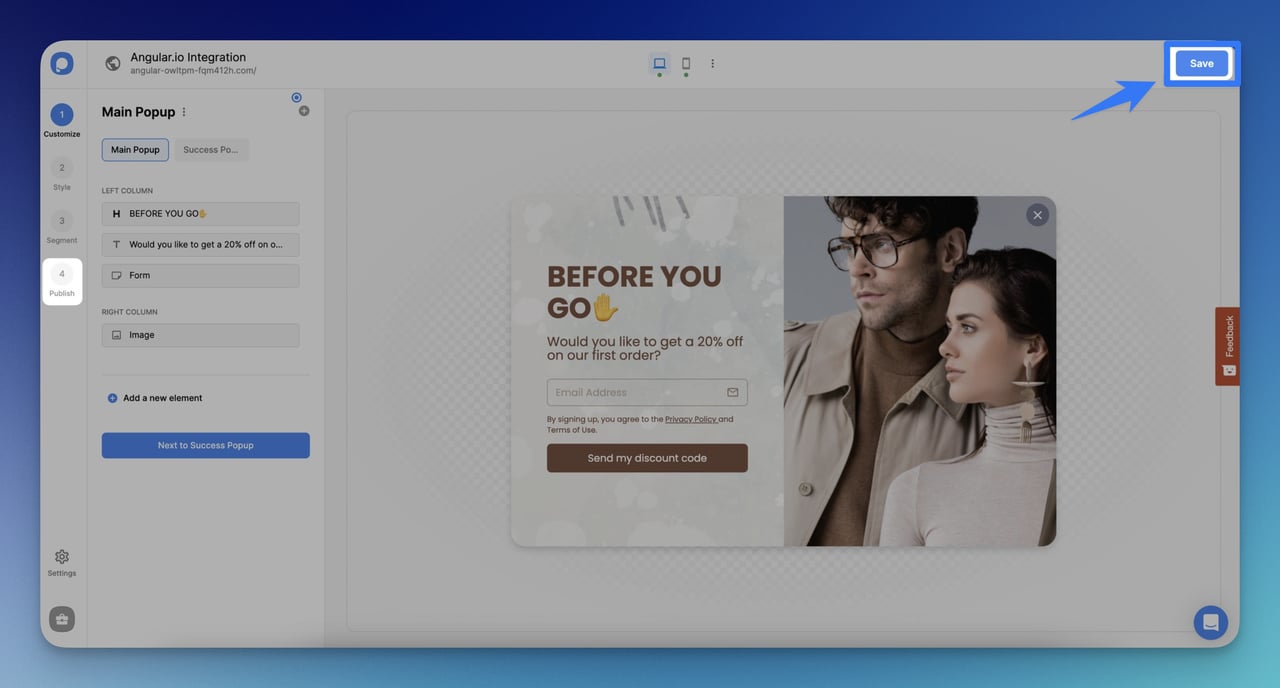
By customizing these popups to fit your brand and target your audience, you can see how your website increases conversions.
We recommend watching the video below to customize your pop-up, style it, and segment your audience with Popupsmart.
Why Choose Angular to Create Popup?
Let's take a look at why you might choose to use Angular to create popups:
- Component-Based Architecture: Angular allows you to create pop-up windows that you can use in different parts of your application and make reusable.
- Two-Way Data Binding: When something changes in your popups, Angular makes sure the screen updates correctly.
- Dependency Injection: Angular quickly provides the tools and data your popups need to run.
- Reactive Forms: If your popups have forms, Angular makes it easy to manage them by checking and submitting data.
- Testing Capabilities: You can quickly test your popups with Angular to make sure they are working correctly.
Wrapping Up
To sum up, we've compiled four different ways, each offering its unique library, from Angular Materials to Ng-bootstrap and PrimeNG.
We've also included Popupsmart, a code-free popup builder for those with limited coding skills.
Whether you're an experienced developer or a beginner, Angular offers a variety of options to improve your website's interactivity and overall user experience.
Frequently Asked Questions
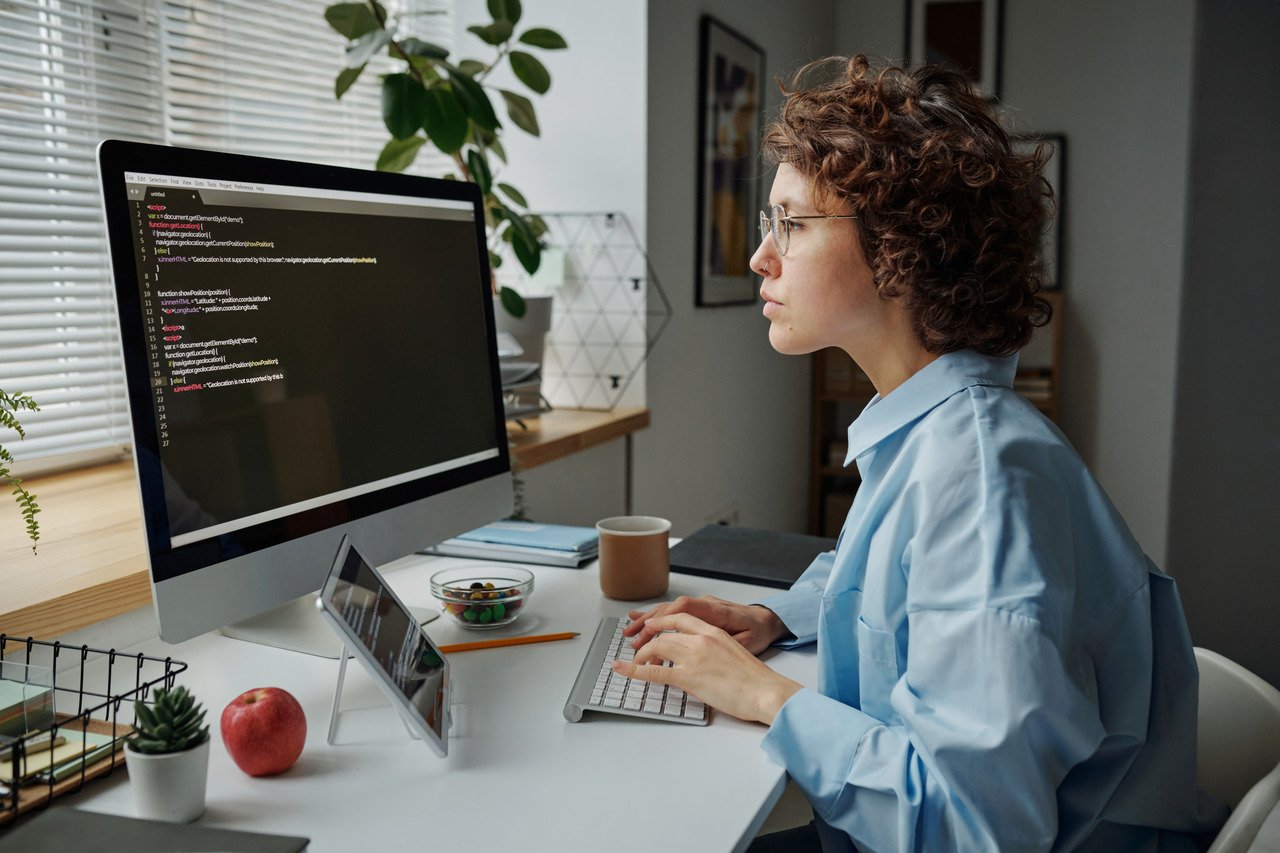
Can I Create Custom Animations for Popups in Angular?
Yes, you can, first, you need to set up Angular animations in your project by importing BrowserAnimationsModule into your app.module.ts file.
Then add effects to your animation using the @angular/animations package.
Apply the animation to your popup element in your component's template using the [@animationName] syntax.
To control when the animation plays, use Angular functions like ngIf or ngClass to change the animation state.
Also, with Popupsmart, you can easily create animated popups without implementing Angular animations manually.
Here's a document on how to do it: How to Add Lottie's Animation to a Popup?
What is the Difference Between a Modal Dialog and a Popup in Angular?
In Angular and web development, the terms "modal dialog" and "popup" are often used as synonyms.
But they can have slight differences, depending on the context.
Modal dialog boxes are like unique pop-ups that prevent you from doing anything else on the web page until you interact with them.
The term “pop-up” is more of a general term that includes many different things on a web page.
How Can I Track User Interactions with Popups in Angular?
You can use event listeners and website analytics tools to monitor user interactions with popups in Angular.
Trigger events that record those actions when a user interacts with the popup.
You can then use Google Analytics to collect and analyze the data.
In addition to event listeners and website analytics tools, popup builders like Popupsmart offer built-in analytics data.
You can easily monitor users' interactions with your popups and gather insights directly from the Popupsmart platform.
This will help you track how users interact with your pop-ups, such as conversion rates and which popups are most effective.