What is React Popup?
A react popup is a small window or box that appears on top of your main web page when a certain action occurs, like clicking a button or hovering over an image.
These popups are commonly used to show important messages, forms, or additional content without moving the user to a new page.
React, a popular JavaScript library used to build user interfaces, allows developers to create popups easily using something called components.
Think of components like building blocks that can be reused in different parts of a website. A popup is one of these reusable blocks.
Here’s a breakdown of what a React popup does:
- Appears on User Interaction: A popup can be set to appear when the user clicks a button, hovers their mouse, or performs another action. For example, if you click a "Sign Up" button, a small form can popup for you to enter your details.
- Keeps You on the Same Page: Instead of redirecting you to another page, a popup opens on top of the current content. This means you can interact with it without losing your place on the website.
- Shows Important Content: A popup can display many things, like alerts, forms, images, or extra information. For example, a store might use a popup to offer a discount code when you visit their website.
- Customizable: In React, you can easily change how the popup looks. Developers can modify its size, color, position, and even add animations to make it more engaging.
How to Create a Popup with React in 4 Steps?
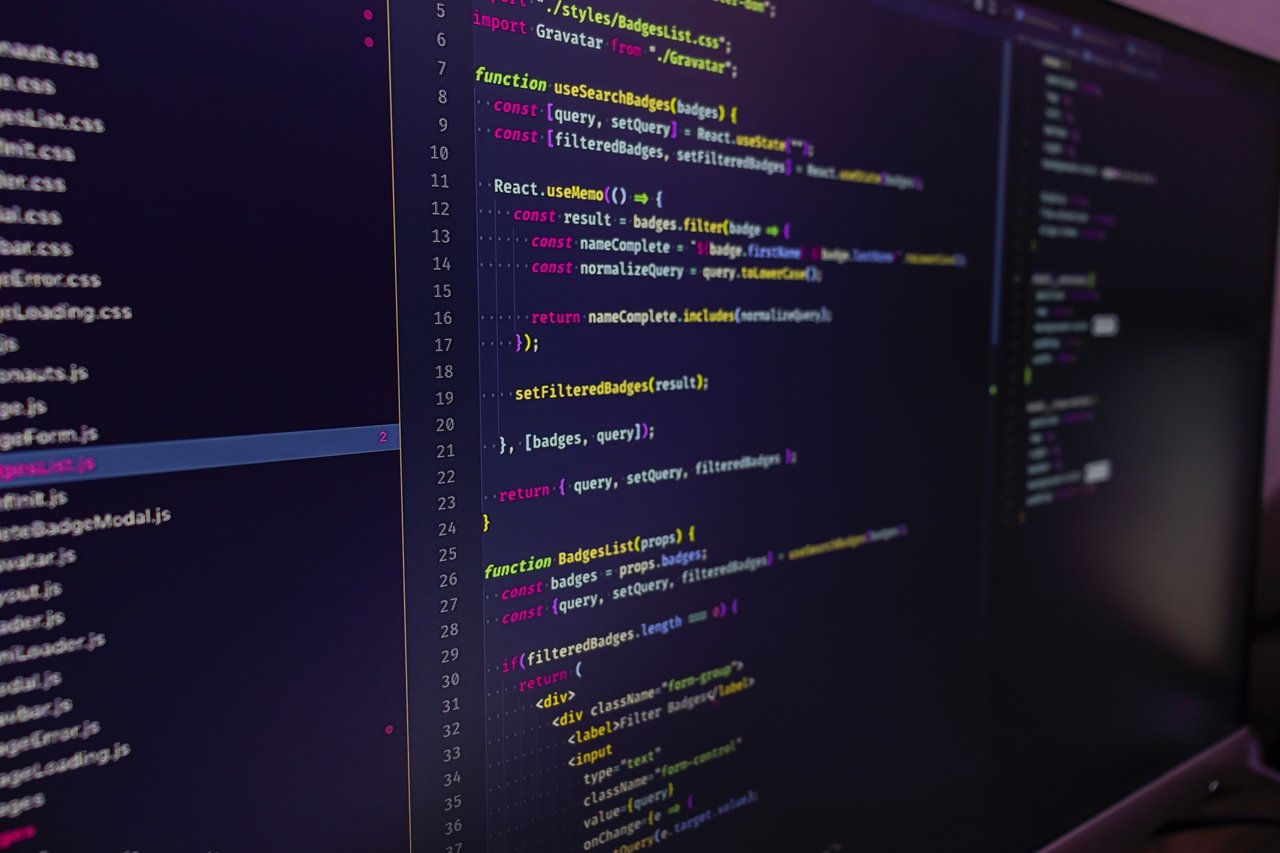
There are different ways to create a popup with React. We will show you how you can create customizable React popups. You can create your own popup for your website by looking at these solutions.
Step 1: Install Required Libraries
To simplify popup creation in React, we’ll use the reactjs-popup package. This package is lightweight and provides customizable options for creating different types of popups.
To install reactjs-popup with npm or yarn, run the following commands in your terminal:
npm install reactjs-popup
or
yarn add reactjs-popup
Step 2: Create a Basic Popup in React
Once you’ve installed the necessary package, let’s create a basic popup. In this example, we’ll create a simple popup that appears when a button is clicked.
import React from 'react';
import Popup from 'reactjs-popup';
import 'reactjs-popup/dist/index.css';
const MyPopup = () => (
<Popup trigger={<button>Open Popup</button>} position="right center">
<div>Popup content here!</div>
</Popup>
);
export default MyPopup;
In the code above, we used the Popup component from reactjs-popup.
The trigger attribute specifies the element that will open the popup, in this case, a button.
The position attribute controls where the popup appears relative to the trigger (e.g., "right center").
When you add this code, your popup will look like this:
Example:
- A button labeled "Open Popup" will appear on your webpage.
- When you click the button, a small box (the popup) will appear next to the button, showing the text "Popup content here!".
Step 3: Customizing the Popup
Customization is an essential part of creating an effective popup in React. You can modify your popup’s width, font size, text alignment, background, padding, and more.
Let’s customize the popup using CSS:
import './MyPopup.css';
const MyPopup = () => (
<Popup
trigger={<button>Open Custom Popup</button>}
position="right center"
contentStyle={{
width: '300px',
padding: '20px',
backgroundColor: '#f1f1f1',
textAlign: 'center',
}}
>
<div>Custom Popup Content</div>
</Popup>
);
export default MyPopup;
When you add this code, your customized popup will look like this:
Example:
- The popup’s width will be 300px, with 20px padding around the text.
- The background color of the popup will be a light gray (#f1f1f1), and the text inside will be center-aligned.
Step 4: Creating Different Types of Popups in React
React allows you to create various types of popups depending on your needs, such as:
- Modal Popup: For displaying important information.
- Prompt Popup: For gathering input from users.
- Notification Popup: For alerting users to important actions.
Example: Creating a Prompt Popup
Prompt popups are useful when you want to collect information from users, such as names or email addresses.
Here’s an example of how to create a prompt popup with React:
import React, { useState } from 'react';
import Popup from 'reactjs-popup';
const PromptPopup = () => {
const [name, setName] = useState('');
return (
<Popup trigger={<button>Enter Name</button>} position="right center">
<div>
<label>Your Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<p>You entered: {name}</p>
</div>
</Popup>
);
};
export default PromptPopup;
When you add this code, your prompt popup will look like this:
Example:
- A button labeled "Enter Name" will appear.
- When clicked, a popup will appear with an input field where users can type their name.
- The name entered will be displayed inside the popup in real-time.
Use Popup Builder Popupsmart for Your Website
If you don't want to deal with coding with React JS, you can also create popups with Popupsmart. Popupsmart is a no-code popup builder, which is easy to use and free!
Popupsmart assists you while creating campaign-driven popups for your website. You can use different features of Popupsmart while creating your popup. In addition, there are many layouts you can choose from for your popup.
Also, you can set your target audience to reach out to your visitors properly.
Let's look at how you can create a popup with Popupsmart step by step.
First, you need to sign up if you haven't already. Then, go to the dashboard and click on the "New Campaign" part.
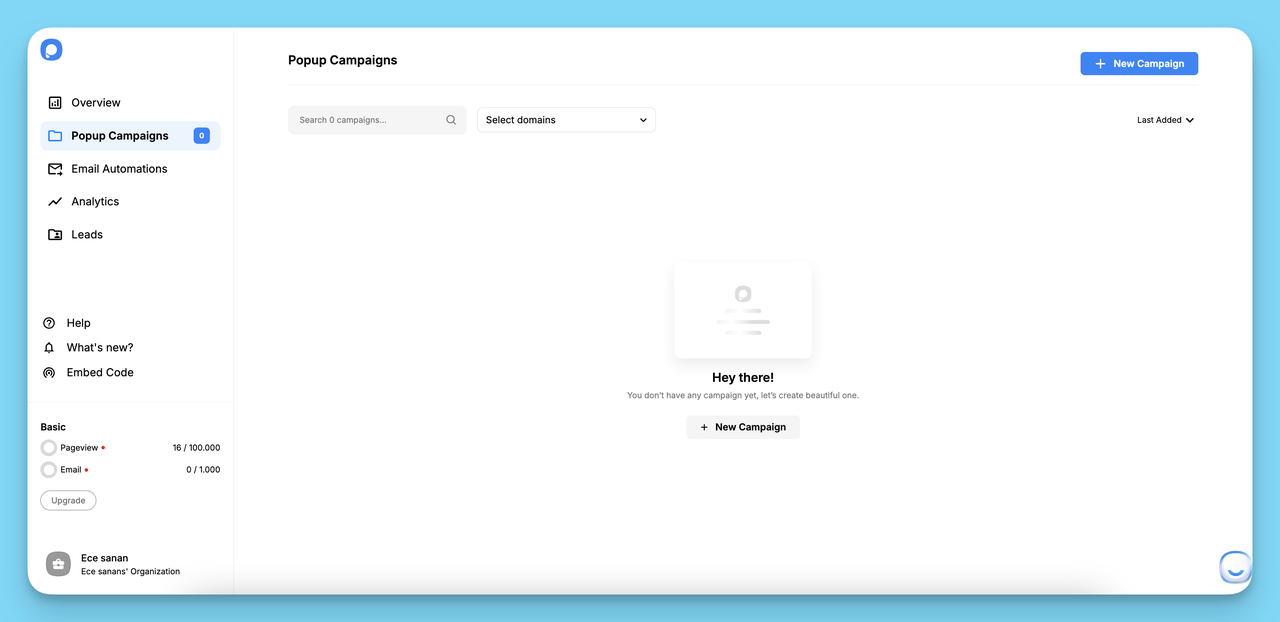
Secondly, you need to select a template that fits your business objective. We have many ready-to-use popup templates that are suitable for different business objectives. By exploring them, you can choose a template for the various aims of your business.

With popups created with Popupsmart, you can:
- Grow your email list and target your visitors.
- Show an announcement of your products and services to your customers.
- Comply with cookie laws and take your visitors' consent for storing cookies.
- Increase phone calls and build stronger relationships with your visitors in an old-fashioned way.
- Promote your products and services by presenting special offers to your audience.
- Collect form submissions to gather personal information and data of your visitors to enhance their user experience.
For this popup creation guide, we'll use a template designed to collect feedback.
After you choose your template, the popup builder screen will show up. In the "Customize" section, where you can play with your popup independently! You can customize your layout’s position, size, color palette & fonts to design your popup more suitable for your website.
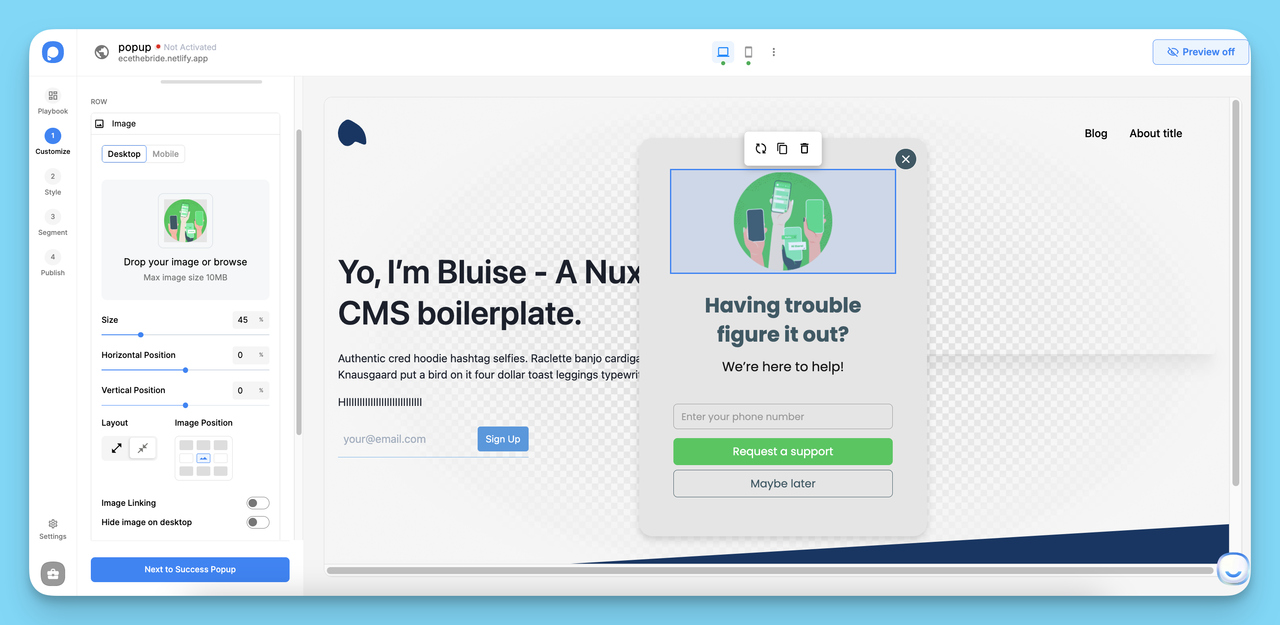
You can edit a popup’s image, text, and input fields according to your business as well.
Make sure you add CTA phrases to your popup so your audience can interact with your popup! Also, customizing your popup's layout according to your website's overall style and personality can be useful.
You can personalize each element you add to your popup to make it more engaging. By clicking on the elements of a popup’s block, you can customize its style according to your needs!

When you have finished customizing your popup and made sure that it looks remarkable, go to the “Target” section.
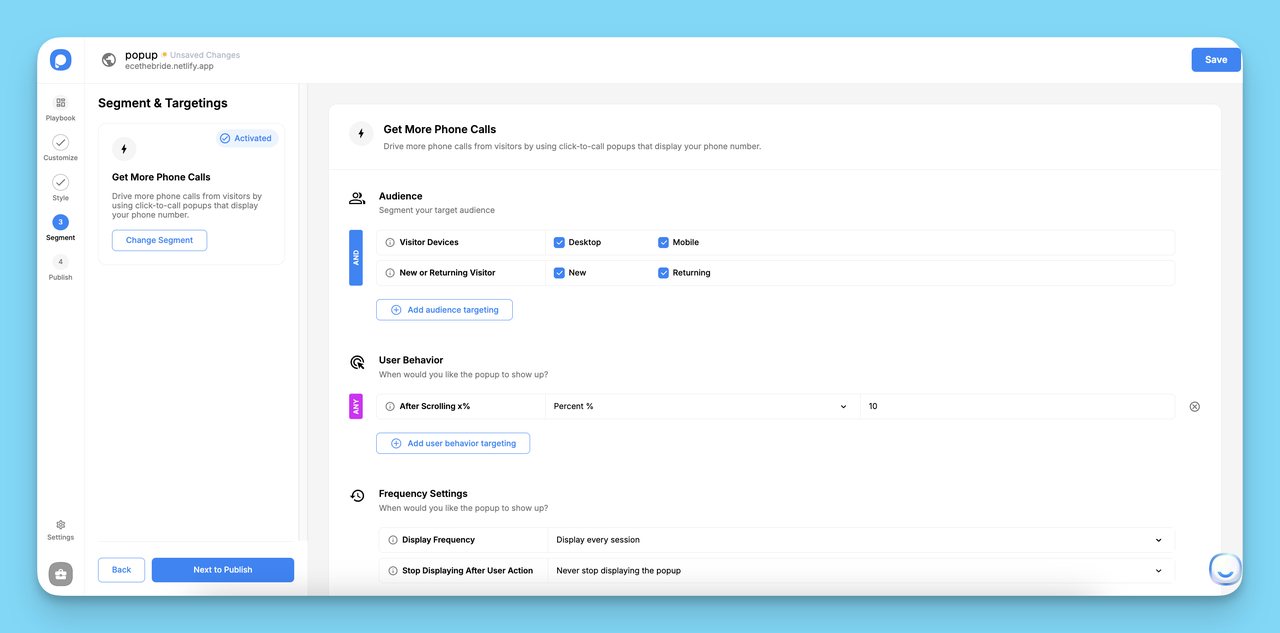
You can optimize the targeting options of your popup in the "Target" section.
There are different targeting options that you can choose according to your business objective.
By setting triggers on the “Audience,” “User Behavior,” and “Frequency Settings” parts, you can build an advanced popup with innovative targeting features to reach your visitors properly.
When you are done with this part, click on the "Save" button and go to the “Settings” part.
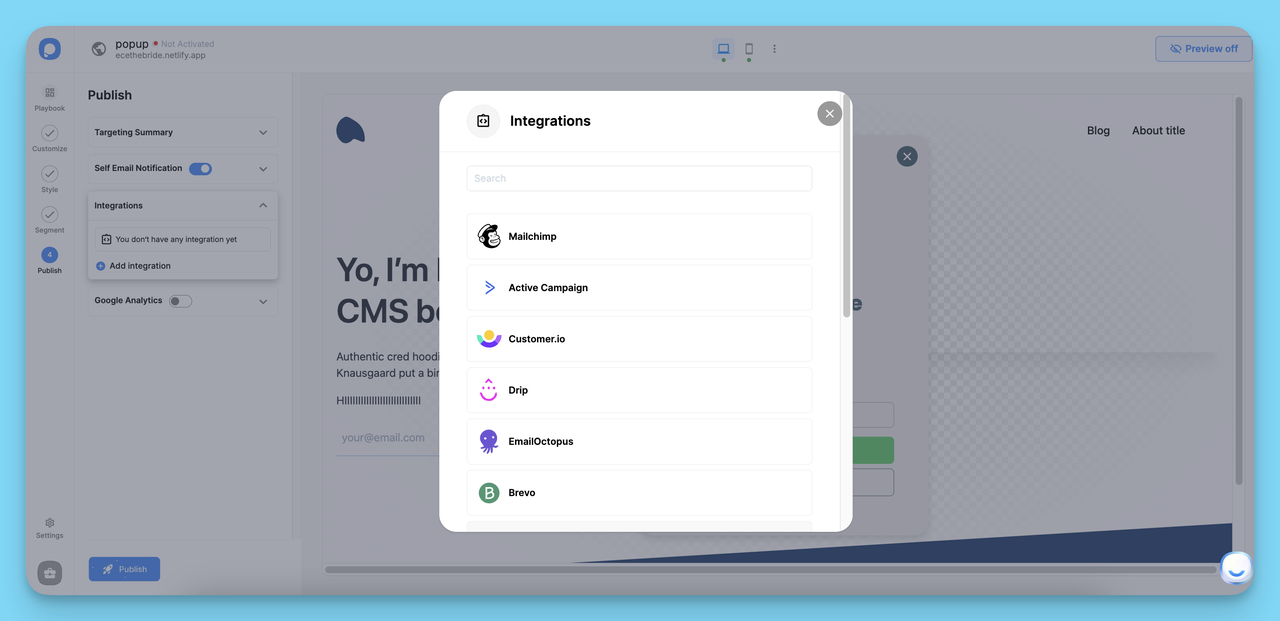
In the "Settings" section, you can see that it is possible to integrate your campaigns with more than 60 apps. Also, you can integrate Popupsmart with Google Analytics and set up autoresponder email notifications on this part.
Click on the "Save & Publish" buttons when you are all set and ready to go!
When you finish creating your popup, add the embed code of your popup to your website's code injection part. You can easily add it before the closing </body> tag. Besides, you can add popups with Google Tag Manager to your website.
You can see the Popupsmart embed code when you click on the "Publish" button.
You can verify your website from this modal and publish your engaging popup!
Very easy, right? Now you can create your own popup to collect feedback, promote your products or services and increase conversions!
Popupsmart integrates with many marketing tools and websites such as Mailchimp, HubSpot, Shopify, Sendinblue, and Squarespace; which makes the digital marketing process of companies even better.
Wrap Up

Creating popup windows with React, which is an open-source JS library, is possible and easy to adapt. As we have explained, adding a popup to your website by using React can be simple if you are familiar with the Javascript coding language. You can install React JS to your website and form your modal popups.
It is possible to customize certain elements of a popup such as width, background, font size, and so on as well. Thanks to different customization options, you can form your own popup to achieve your business goals.
On the other hand, not everyone has to deal with writing codes and adding more codes to customize their popup. You can use our popup builder Popupsmart, which has a free-to-use pricing plan.
We hope you liked reading about this topic and learned how you could create a popup easily with React and Popupsmart. Let us know which popup solution you decide to choose for your website. :-)
Check out these blog posts as well: